
Godot is a good and free open-source game engine that can be used for personal and commercial projects of any purpose, not just games. To illustrate the power of this game engine, let us build a simple game environment for a player that can navigate around on a platform.
The goal here is just to create a player or sprite that can move left or right and jump around a platform environment. It is not a complete game but this tutorial guide may serve as a taste of what working with the Godot game engine can be like.
Readers should at least learn about the basics of the Godot game engine interface. Feel free to use this post to refer back and review Godot interface basics.
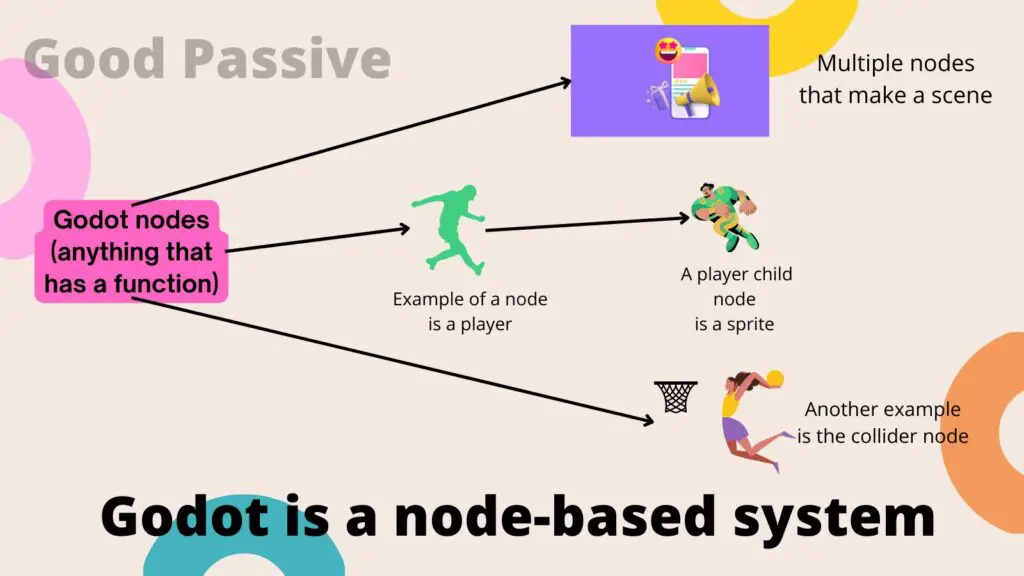
Just download and double-click to run the Godot game engine file.
Start a new project with a new empty folder.
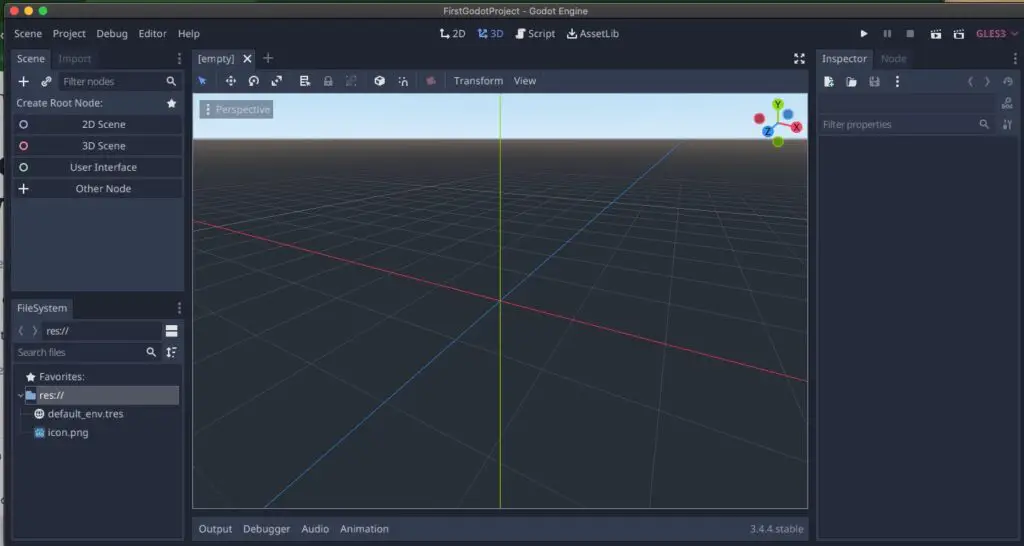
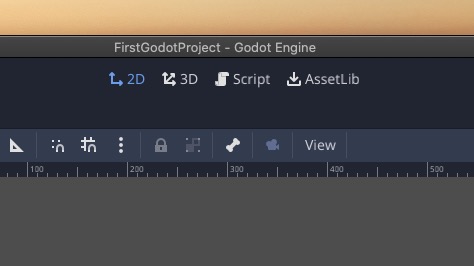
Click on 2D Scene
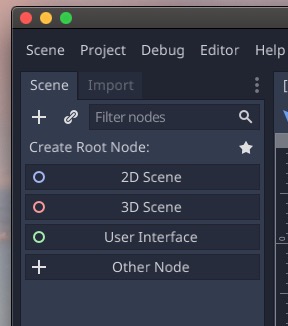

Notice the Inspector on the right where we can change the options e.g Transform and visibility
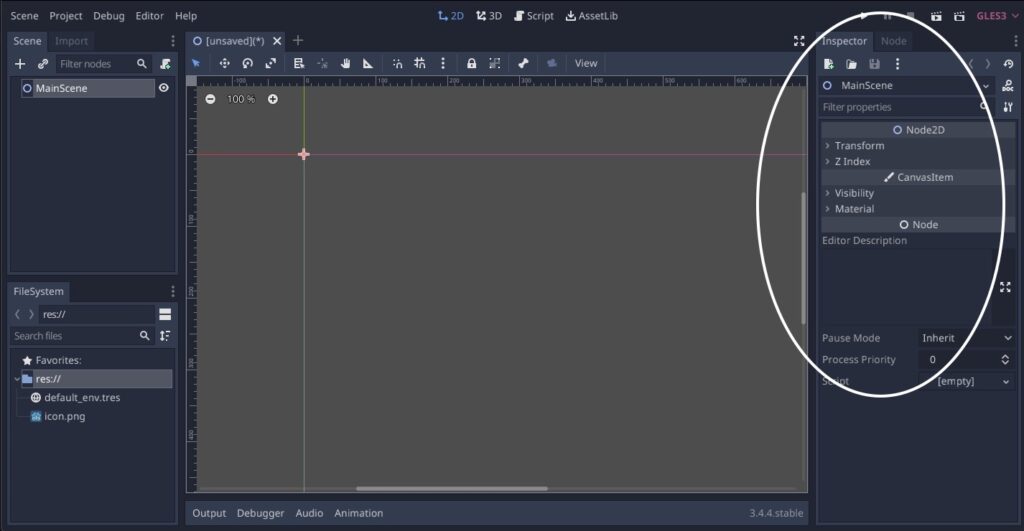
Type CMD+S (or Ctrl+s for Windows) to save the scene e.g. MainScene.tscn.

The filesystem is where we can have an overview and start importing assets into the game.
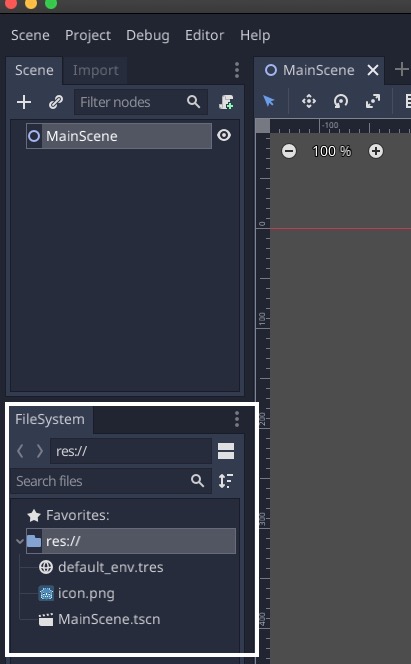
We can also click 3D from the top to view it in 3D. Next to it is the Script part where we put in the codes for the nodes or assets.
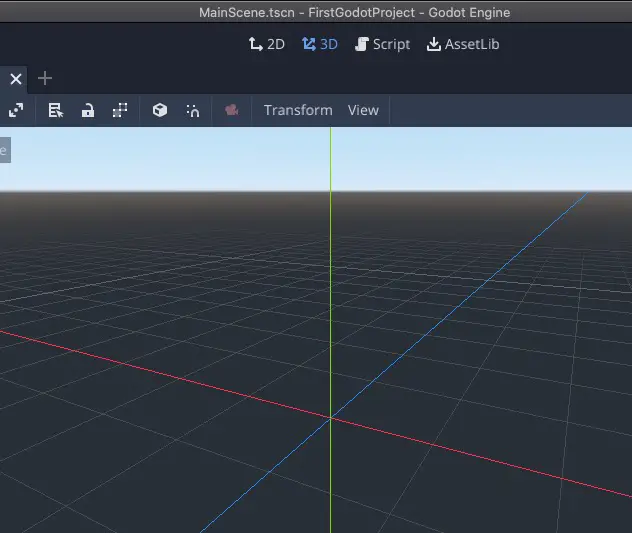
The middle or right mouse click can move the axis point around.
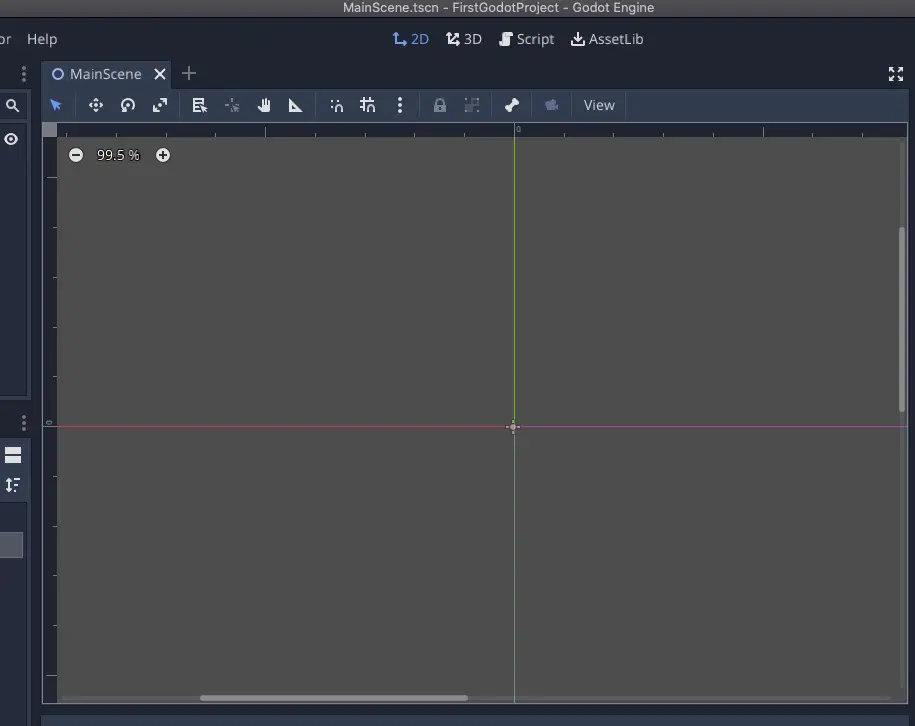
On the top-right are the buttons to play, pause, and stop the game. We can also play the scene with the action-cut icon. The last is the custom scene for selecting a scene to launch.

Let us start importing some assets into the game.
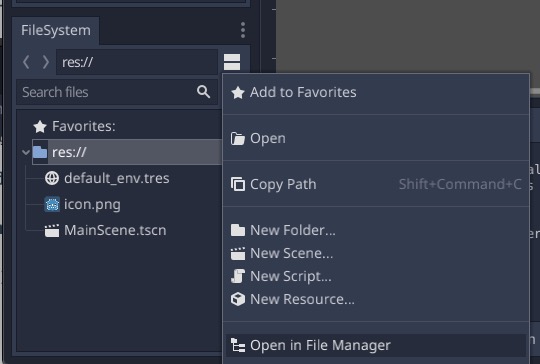
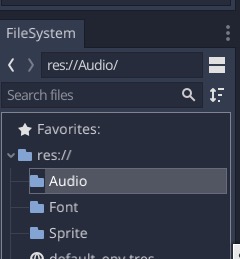
Start gathering some assets for your game e.g free assets from Kenney.nl. And import these assets into your asset folders,
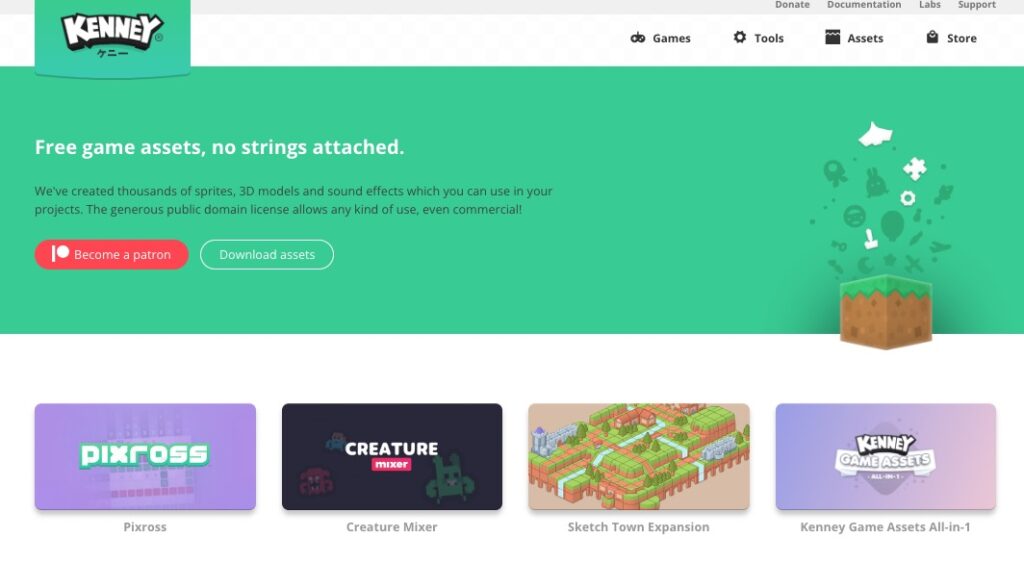
For example, we need to collect a player sprite (idle, jump, first walking, second walking poses), a robot sprite, a coin or reward sprite, a sound for collecting the reward, and font or two for the game. For the purpose of this simple tutorial, just get one sprite image and one tile.
All can be found on Kenney for free.


Next, we will create a player in a new scene.
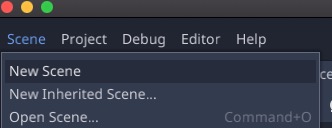
For a player sprite, we need to apply physics to it. Click on Other Node -> Kinematics2DBody.
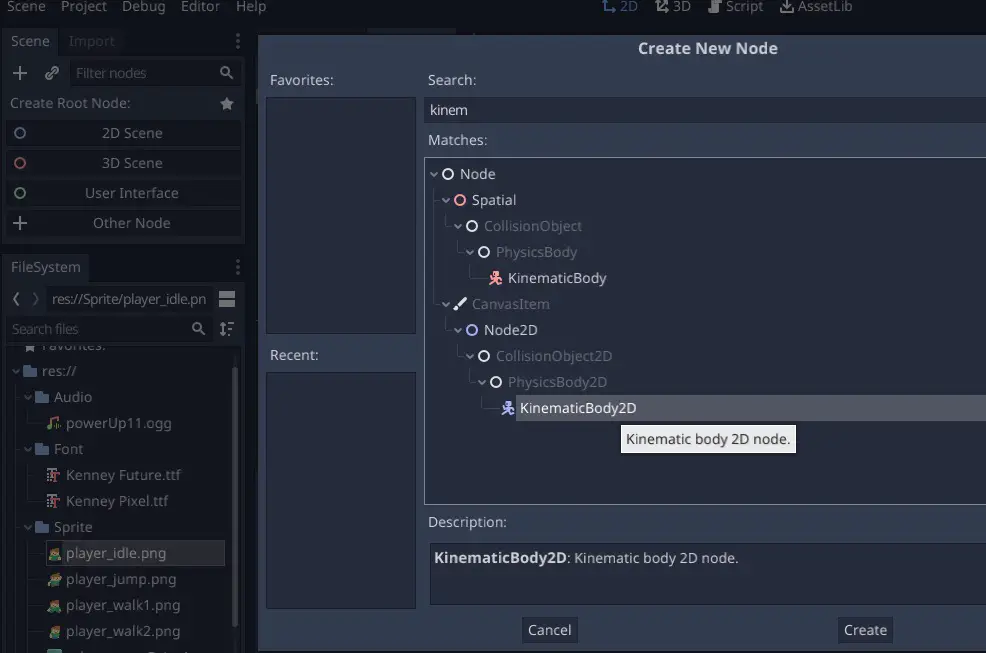
We will leave the Inspector physics settings for this Kinematics2DBody alone for now.

We may double click the word Kinematics2dBody to rename it e.g. Player
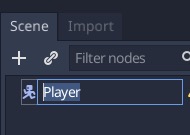
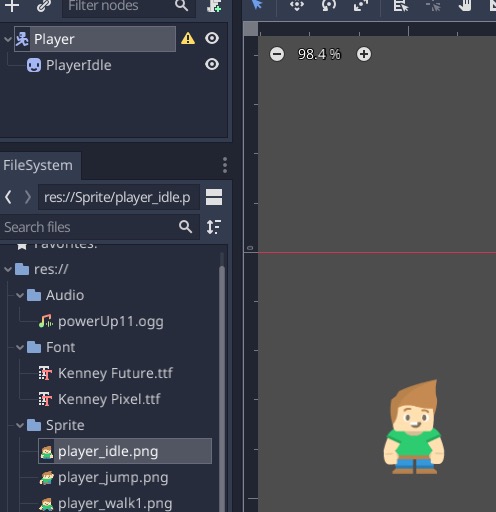
Whatever happens to the parent Player node will apply to all the children nodes.
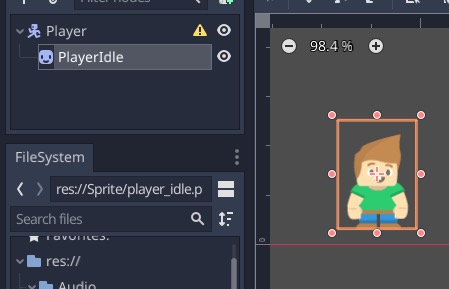




Next, we need to fix the collide node error message. Right now it does not know how it can collide with other objects without a shape.


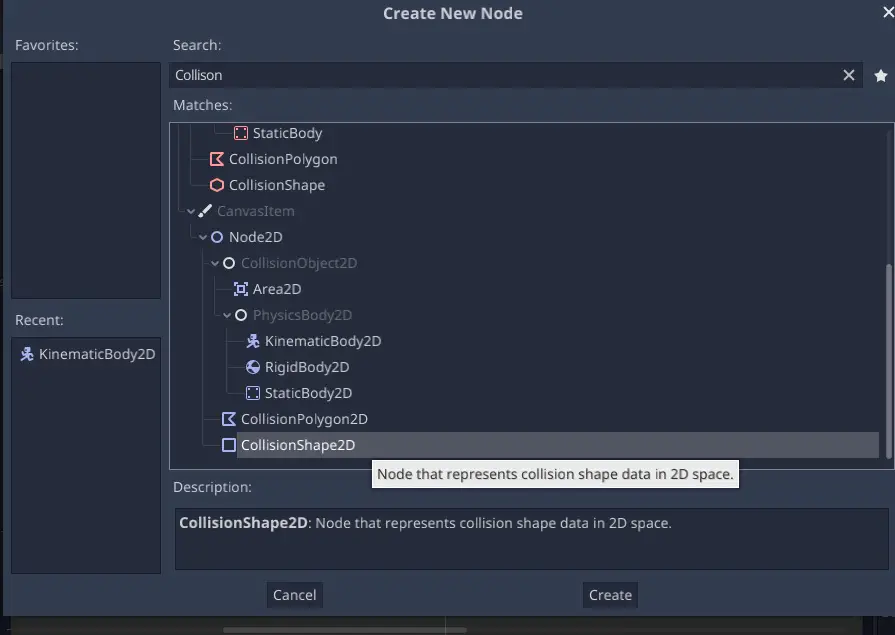
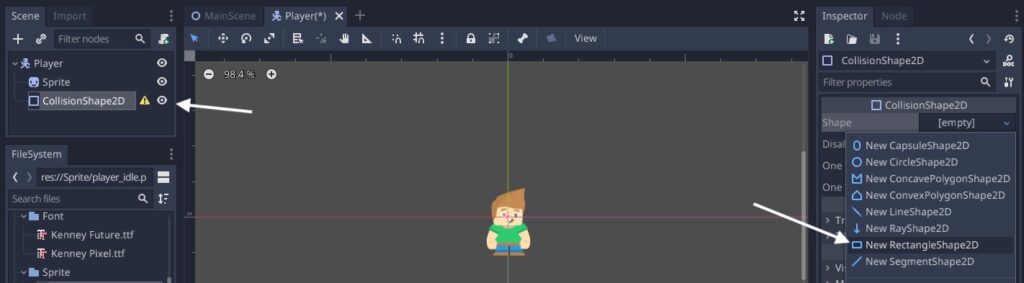



A Player node that is an instance of the Main Scene appears.
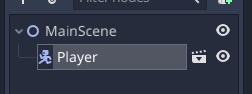
Any changes made on the Player scene will also update on the Player node in the Main Scene

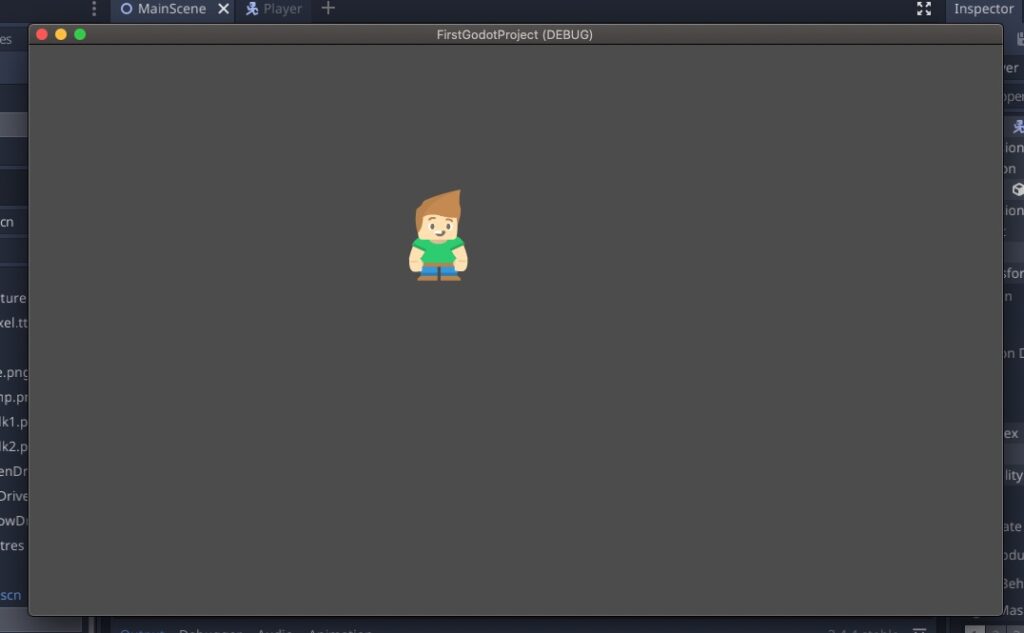
Quit the game with the stop button or close the game window. Congratulations, now you have managed to display a sprite on our Godot game.
Next, we will begin to control the movements of our sprite with scripting.
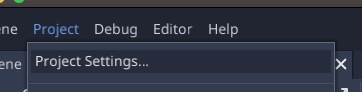
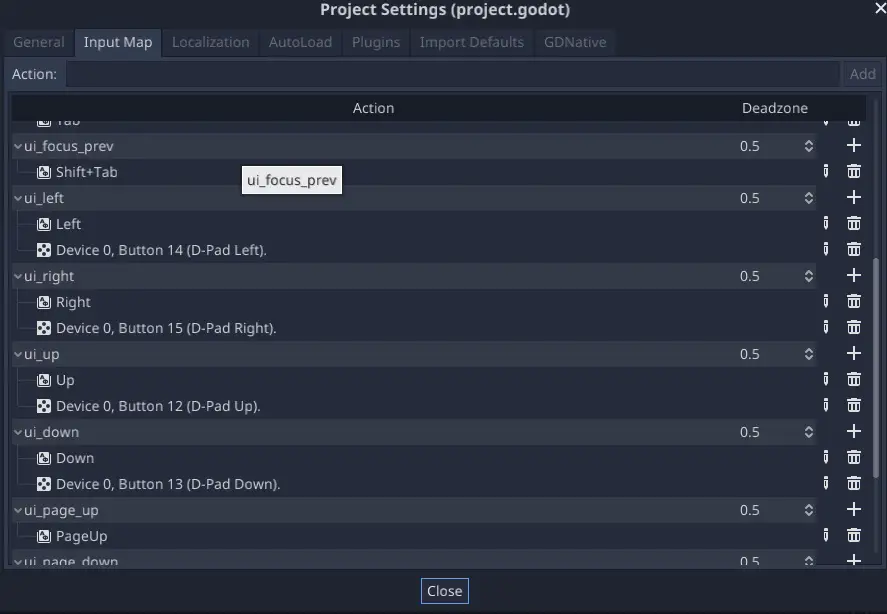
We are interested in 3 inputs: left, right, and jump. Let’s create a new one by typing in the Action box and clicking Add: move_left
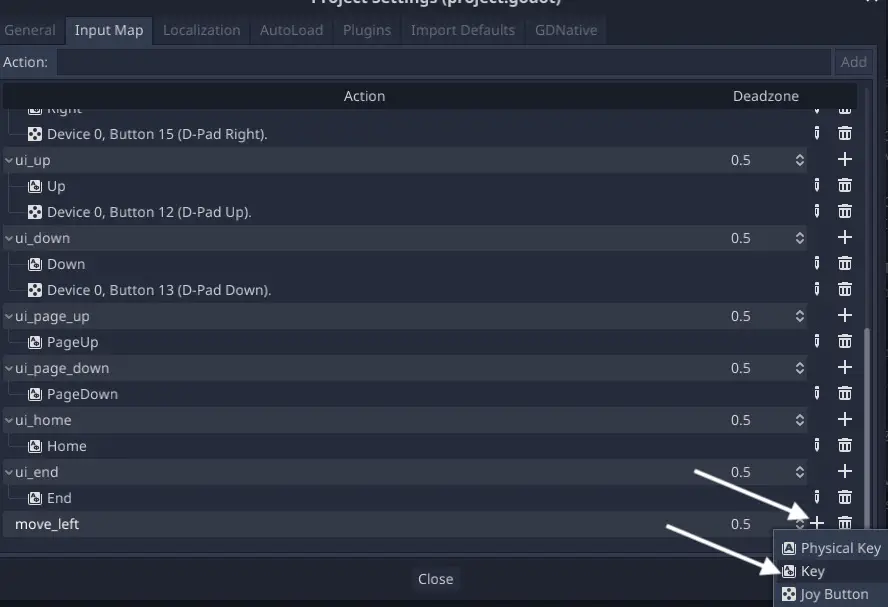
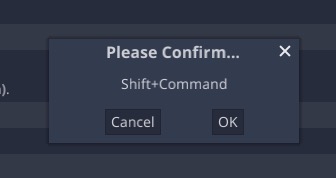
Repeat by creating move_right for the right arrow and jump for the up arrow.


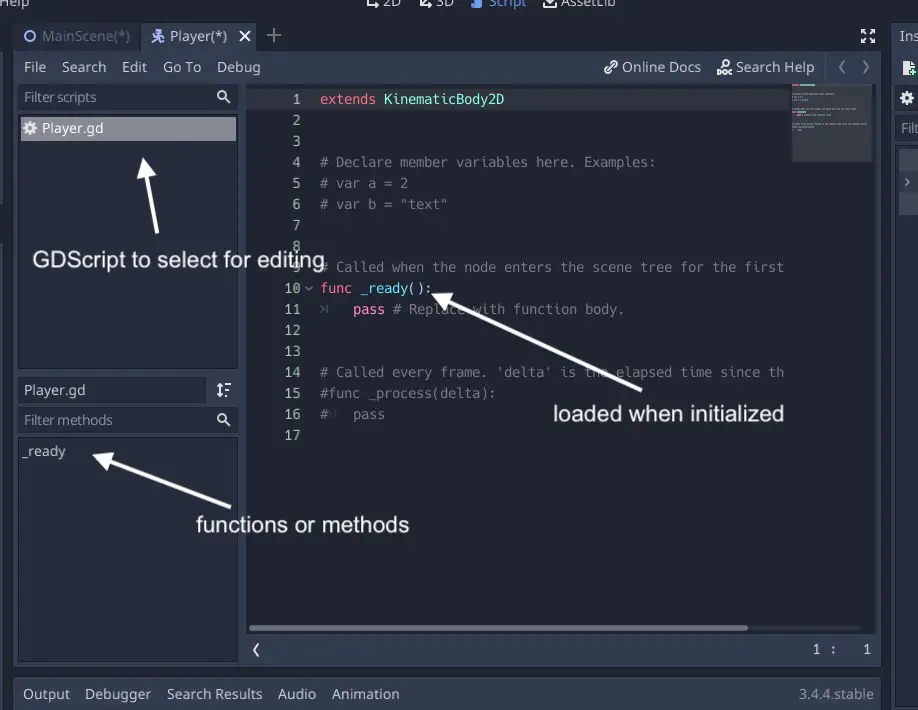
Delete everything except the first line so we can use functions the KinematicBody2D such as move, slide, and collide.

Next, we create different variable types and assign some values.
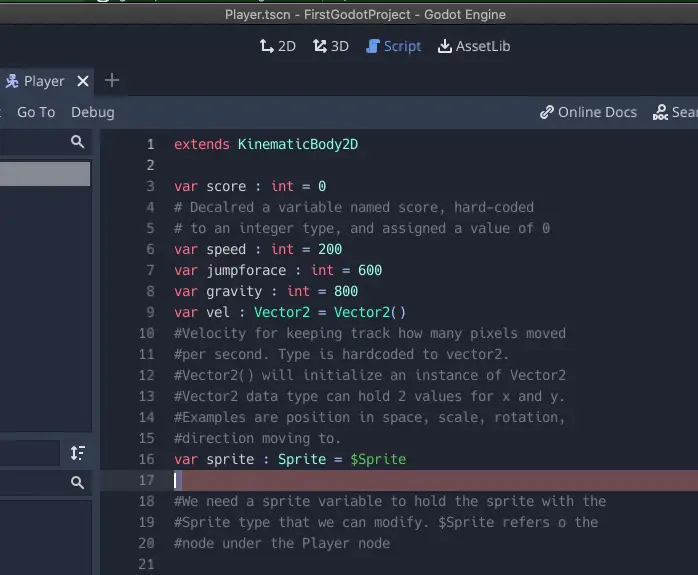
We also create a Sprite date type for our sprite so we can modify values dynamically during the game. For example, we want the sprite to face left when moving left and right when moving right by setting the Flip H (horizontal) value of the Sprite.
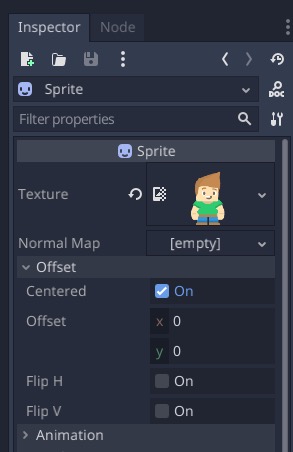
We get an error below the code screen that prompts us to use another function. Now the error is gone. We get the sprite only after it is initialized or ready with the ‘onready’ command.

Next, we will make the sprite move by calling the _physics_process built-in function.
Code the basic physics of the sprite, save and run the game.
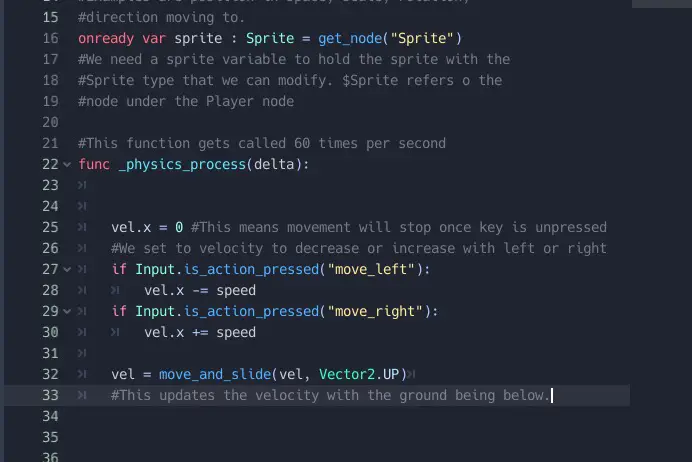

Next, we make it face the direction of the movement.

Save and click play to check.

Next is the application the gravity and the ability to jump.

Note: I amended the variable typo from jumpforace to jumpforce.
Save and click play. We have not applied a ground for the sprite to stand on, so it will fall right down once the game is started.

Next, we will create the tiles for the game.
Create a new scene. Click + Other Node. Select StaticBody2D for creation.
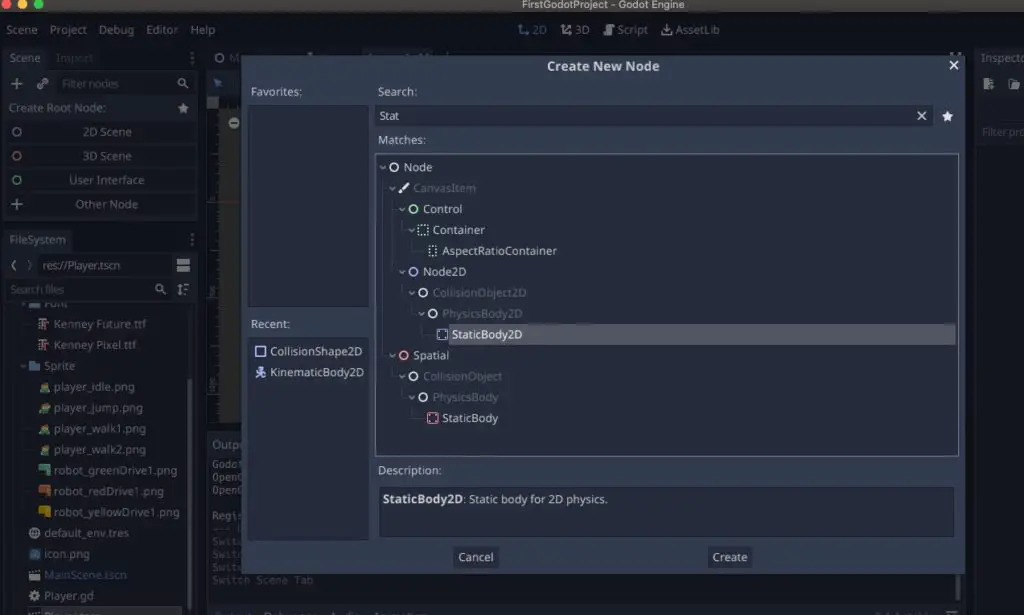
This type of node is static and useful for collision.
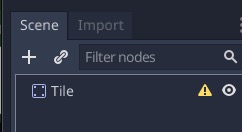

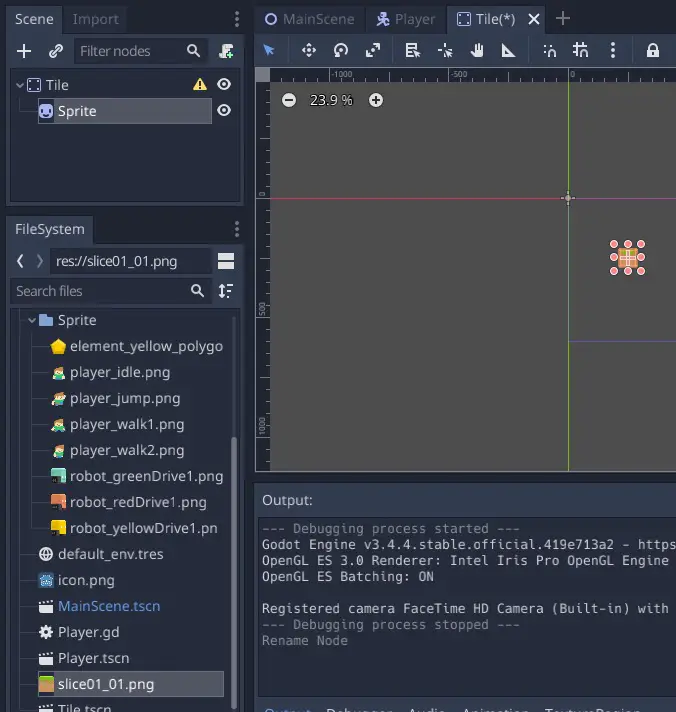

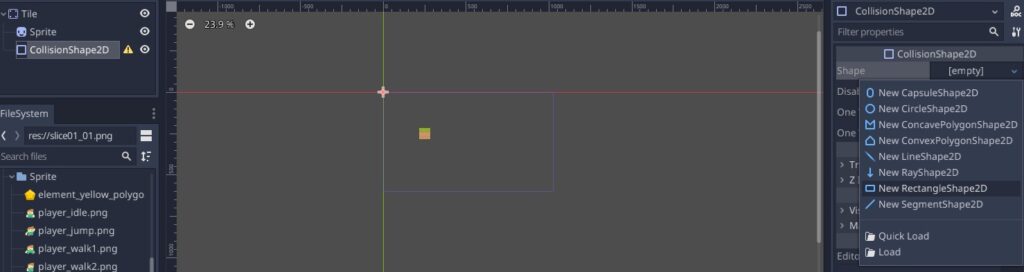
Position both the tile and rectangle collider area to 0 on the x and y-axis with Transform.
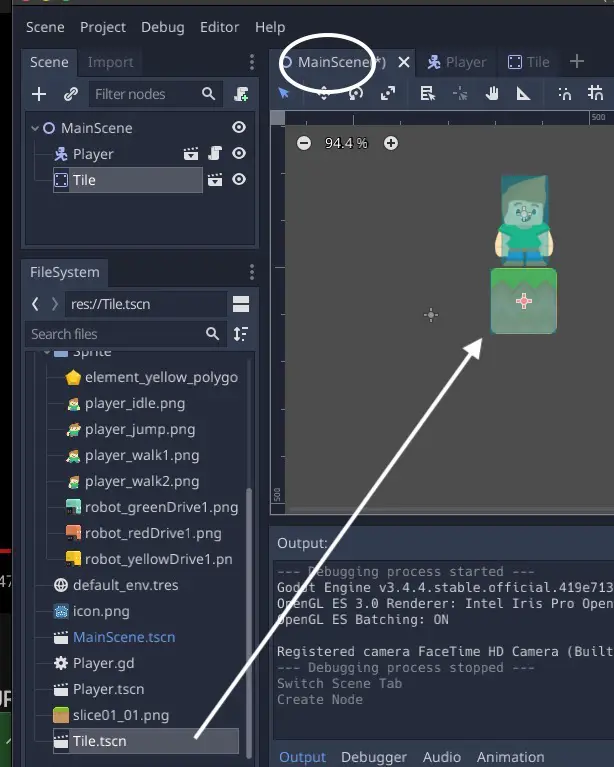
Save and click play.


Sprite will fall if it moves beyond the tile floor.
Now, we can build a platform by duplicating the tile with Ctrl+d or CMD+d and dragging it out with a mouse click.

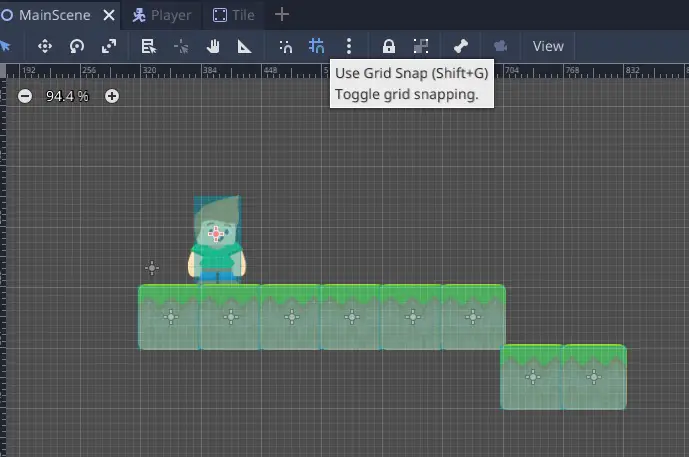
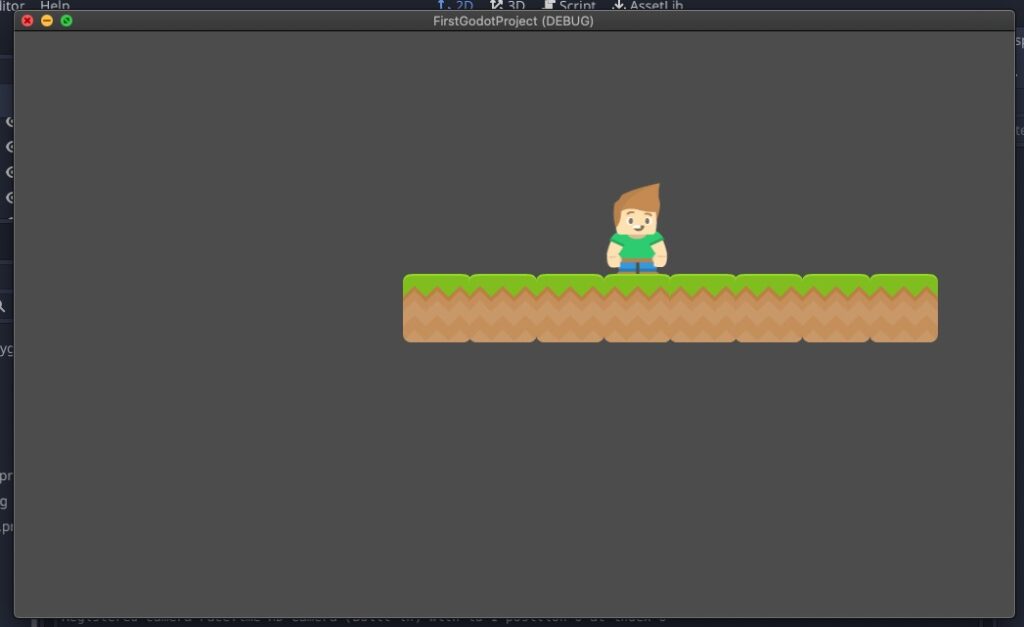

At this point, the player or sprite can move around this little one-scene platform.
What next?
This is far from being a complete game e.g. player can vanish moving beyond the screen, there are no opening welcome screen or ending credits, or lacks any interaction with other objects or nodes. Game development is a long and hard journey, at least for a decent release. And given the extreme high and tough competition out there on various platforms, success is an anomaly.
Conclusion
Godot is a free, lightweight, and stable game engine for creating games. However, building a game will require some learning, and access to support may be important if we get stuck. Another reason I recommend Godot is that they are known for their friendly and nice community.
The hardest part of this tutorial or games development to understand may be the programming physics logic. The version of the Godot used here is v3.4.4 stable. The game engine and all assets used are all released for free use.
One of the problems I realized with learning from free resources maybe they are incomplete on purpose e.g. building a complete game. Rather than learning from one free resource, and then scouting to see if we can find the next level resource, or not. This is not ideal for an arduous project like game development, and definitely without support when we run into problems that will happen.
We can learn from free game development YouTube videos and blogs, however, a more complete and effectively faster learning path is to follow a good and most likely a paid course as well. Our human time and energy are too limited, even free learning resources do not mean it will be worth it.
I believe one of the more complete and comprehensive Godot resources with both the Godot and GDQuest course and community support can be GDQuest’s Learn to Code From Zero With Godot (good for beginners).
Be wholesome.
More Information
What are some Godot or game engine-related posts?